How To Integrate Google Maps Drawing Manager in React 2023
@react-google-maps/api provides a set of functions for implementing Google Maps API. In this tutorial, we will integrate google maps drawing manager into the react project step by step.
1. Let's install create-react-app package.
npm i -g create-react-app
2. Create a new react project
create-react-app react-google-maps-drawing-manager
cd react-google-maps-drawing-manager
3. Install @react-google-maps/api package inside the project.
npm i @react-google-maps/api
4. Create assets/images folder inside the src folder. download remove icon from this link and copy it into the images folder.
5. Create folder components inside the src folder. Inside this folder create map.component.jsx file.
import React, { useRef, useState } from 'react';
import { Autocomplete, DrawingManager, GoogleMap, Polygon, useJsApiLoader } from '@react-google-maps/api';
import deleteIcon from '../assets/images/remove.png';
const libraries = ['places', 'drawing'];
const MapComponent = () => {
const mapRef = useRef();
const polygonRefs = useRef([]);
const activePolygonIndex = useRef();
const autocompleteRef = useRef();
const drawingManagerRef = useRef();
const { isLoaded, loadError } = useJsApiLoader({
googleMapsApiKey: 'Your Google API Key',
libraries
});
const [polygons, setPolygons] = useState([
[
{ lat: 28.630818281028954, lng: 79.80954378826904 },
{ lat: 28.62362346815063, lng: 79.80272024853515 },
{ lat: 28.623585797675588, lng: 79.81490820629882 },
{ lat: 28.630818281028954, lng: 79.80954378826904 }
],
[
{ lat: 28.63130796240949, lng: 79.8170110581665 },
{ lat: 28.623623468150655, lng: 79.81705397351074 },
{ lat: 28.623623468150655, lng: 79.82619494183349 },
{ lat: 28.6313832978037, lng: 79.82619494183349 },
{ lat: 28.63130796240949, lng: 79.8170110581665 }
]
]);
const defaultCenter = {
lat: 28.626137,
lng: 79.821603,
}
const [center, setCenter] = useState(defaultCenter);
const containerStyle = {
width: '100%',
height: '400px',
}
const autocompleteStyle = {
boxSizing: 'border-box',
border: '1px solid transparent',
width: '240px',
height: '38px',
padding: '0 12px',
borderRadius: '3px',
boxShadow: '0 2px 6px rgba(0, 0, 0, 0.3)',
fontSize: '14px',
outline: 'none',
textOverflow: 'ellipses',
position: 'absolute',
right: '8%',
top: '11px',
marginLeft: '-120px',
}
const deleteIconStyle = {
cursor: 'pointer',
backgroundImage: `url(${deleteIcon})`,
height: '24px',
width: '24px',
marginTop: '5px',
backgroundColor: '#fff',
position: 'absolute',
top: "2px",
left: "52%",
zIndex: 99999
}
const polygonOptions = {
fillOpacity: 0.3,
fillColor: '#ff0000',
strokeColor: '#ff0000',
strokeWeight: 2,
draggable: true,
editable: true
}
const drawingManagerOptions = {
polygonOptions: polygonOptions,
drawingControl: true,
drawingControlOptions: {
position: window.google?.maps?.ControlPosition?.TOP_CENTER,
drawingModes: [
window.google?.maps?.drawing?.OverlayType?.POLYGON
]
}
}
const onLoadMap = (map) => {
mapRef.current = map;
}
const onLoadPolygon = (polygon, index) => {
polygonRefs.current[index] = polygon;
}
const onClickPolygon = (index) => {
activePolygonIndex.current = index;
}
const onLoadAutocomplete = (autocomplete) => {
autocompleteRef.current = autocomplete;
}
const onPlaceChanged = () => {
const { geometry } = autocompleteRef.current.getPlace();
const bounds = new window.google.maps.LatLngBounds();
if (geometry.viewport) {
bounds.union(geometry.viewport);
} else {
bounds.extend(geometry.location);
}
mapRef.current.fitBounds(bounds);
}
const onLoadDrawingManager = drawingManager => {
drawingManagerRef.current = drawingManager;
}
const onOverlayComplete = ($overlayEvent) => {
drawingManagerRef.current.setDrawingMode(null);
if ($overlayEvent.type === window.google.maps.drawing.OverlayType.POLYGON) {
const newPolygon = $overlayEvent.overlay.getPath()
.getArray()
.map(latLng => ({ lat: latLng.lat(), lng: latLng.lng() }))
// start and end point should be same for valid geojson
const startPoint = newPolygon[0];
newPolygon.push(startPoint);
$overlayEvent.overlay?.setMap(null);
setPolygons([...polygons, newPolygon]);
}
}
const onDeleteDrawing = () => {
const filtered = polygons.filter((polygon, index) => index !== activePolygonIndex.current)
setPolygons(filtered)
}
const onEditPolygon = (index) => {
const polygonRef = polygonRefs.current[index];
if (polygonRef) {
const coordinates = polygonRef.getPath()
.getArray()
.map(latLng => ({ lat: latLng.lat(), lng: latLng.lng() }));
const allPolygons = [...polygons];
allPolygons[index] = coordinates;
setPolygons(allPolygons)
}
}
return (
isLoaded
?
<div className='map-container' style={{ position: 'relative' }}>
{
drawingManagerRef.current
&&
<div
onClick={onDeleteDrawing}
title='Delete shape'
style={deleteIconStyle}>
</div>
}
<GoogleMap
zoom={15}
center={center}
onLoad={onLoadMap}
mapContainerStyle={containerStyle}
onTilesLoaded={() => setCenter(null)}
>
<DrawingManager
onLoad={onLoadDrawingManager}
onOverlayComplete={onOverlayComplete}
options={drawingManagerOptions}
/>
{
polygons.map((iterator, index) => (
<Polygon
key={index}
onLoad={(event) => onLoadPolygon(event, index)}
onMouseDown={() => onClickPolygon(index)}
onMouseUp={() => onEditPolygon(index)}
onDragEnd={() => onEditPolygon(index)}
options={polygonOptions}
paths={iterator}
draggable
editable
/>
))
}
<Autocomplete
onLoad={onLoadAutocomplete}
onPlaceChanged={onPlaceChanged}
>
<input
type='text'
placeholder='Search Location'
style={autocompleteStyle}
/>
</Autocomplete>
</GoogleMap>
</div>
:
null
);
}
export default MapComponent;
Note:- Don't forget to update your Google API Key.
6. Open the App.js file and make the following changes.
import React from "react";
import "./App.css";
import MapComponent from "./components/map.component";
function App() {
return (
<div className="App">
<h1>React Google Maps - DrawingManager</h1>
<MapComponent/>
</div>
);
}
export default App;
7. (Optional) if you are using react v18 either handle the component mounting issue during the dev environment or remove StrictMode from index.js
Ref:- App mounting twice in dev mode · Issue #12363 · facebook/create-react-app (github.com)
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
8. Finally start the project.
npm start
open http://localhost:3000 in the browser.
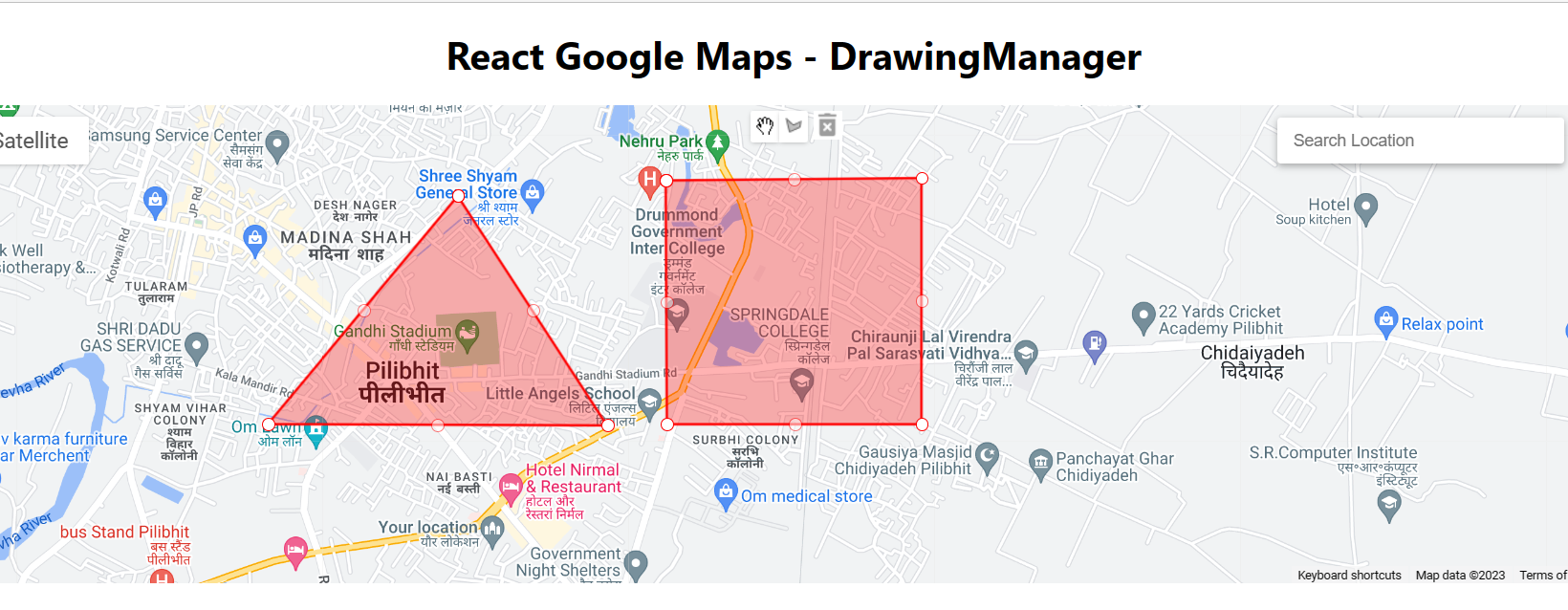
Checkout my full react-google-maps-drawing-manager example.
https://github.com/ultimateakash/react-google-maps-drawing-manager
If you facing any issues. don't hesitate to comment below. I will be happy to help you.
Thanks.
Leave Your Comment