How To Integrate Google Maps Drawing Manager in Angular 2023
Angular Google Maps (AGM) provides a set of functions for implementing Google Maps API. In this tutorial, we will integrate google maps drawing manager into the angular project step by step.
1. Let's install @angular/cli package.
npm install -g @angular/cli
2. Create a new angular project.
ng new angular-google-maps-drawing-manager
cd angular-google-maps-drawing-manager
3. Install @agm/core and @agm/drawing packages.
npm install @agm/core@1.1.0 @agm/drawing@1.1.0
Note:- Latest version of @agm/core and @agm/drawing has a few bugs also it's in the beta state. so install version 1.1.0.
4. Install @types/googlemaps package.
npm install --save-dev @types/googlemaps
5. Open tsconfig.app.json and update "types" property to ["googlemaps"] inside compilerOptions.
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/app",
"types": ["googlemaps"]
},
"files": [
"src/main.ts"
],
"include": [
"src/**/*.d.ts"
]
}
Note:- If you got "Generic type ‘ModuleWithProviders‘ requires 1 type argument(s)” error. then add "skipLibCheck": true inside compilerOptions.
{
"extends": "./tsconfig.json",
"compilerOptions": {
"outDir": "./out-tsc/app",
"types": ["googlemaps"],
"skipLibCheck": true
},
"files": [
"src/main.ts"
],
"include": [
"src/**/*.d.ts"
]
}
6. Open app.module.ts and import AgmCoreModule
import { AgmCoreModule } from '@agm/core';
import { AgmDrawingModule } from '@agm/drawing';
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AgmCoreModule.forRoot({
apiKey: 'Your Google API Key'
libraries: ['drawing']
}),
AgmDrawingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Note:- Don't forget to update your Google API Key.
7. Open styles.css file and add this css.
agm-map {
height: 400px;
}
Note:- It is really important that you define the height of agm-map. Otherwise, you won't see a map on the page.
8. Create images folder inside the assets folder. download remove icon from this link and copy it into the images folder.
9. Open app.component.ts and add this code.
import { Component, QueryList, ViewChildren } from '@angular/core';
import { AgmMap, AgmPolygon, ControlPosition, LatLng, LatLngLiteral } from '@agm/core';
import { DrawingControlOptions } from '@agm/drawing/google-drawing-types';
import { OverlayType } from '@agm/drawing';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
@ViewChildren(AgmPolygon) public polygonRefs!: QueryList<AgmPolygon>;
mapRef!: AgmMap;
zoom: number = 15;
lat: number = 28.626137;
lng: number = 79.821603;
activePolygonIndex!: number;
drawingMode: any = null;
drawingControlOptions: DrawingControlOptions = {
position: ControlPosition.TOP_CENTER,
drawingModes: [
OverlayType.POLYGONE
]
}
polygonOptions = {
fillOpacity: 0.3,
fillColor: '#ff0000',
strokeColor: '#ff0000',
strokeWeight: 2,
draggable: true,
editable: true
}
deleteIconStyle = {
cursor: 'pointer',
backgroundImage: 'url(../assets/images/remove.png)',
height: '24px',
width: '24px',
marginTop: '5px',
backgroundColor: '#fff',
position: 'absolute',
top: "2px",
left: "52%",
zIndex: 99999
}
polygons: LatLngLiteral[][] = [
[
{ lat: 28.630818281028954, lng: 79.80954378826904 },
{ lat: 28.62362346815063, lng: 79.80272024853515 },
{ lat: 28.623585797675588, lng: 79.81490820629882 },
{ lat: 28.630818281028954, lng: 79.80954378826904 }
],
[
{ lat: 28.63130796240949, lng: 79.8170110581665 },
{ lat: 28.623623468150655, lng: 79.81705397351074 },
{ lat: 28.623623468150655, lng: 79.82619494183349 },
{ lat: 28.6313832978037, lng: 79.82619494183349 },
{ lat: 28.63130796240949, lng: 79.8170110581665 }
]
]
onLoadMap($event: AgmMap) {
this.mapRef = $event;
}
onOverlayComplete($overlayEvent: any) {
this.drawingMode = this.drawingMode === null ? '' : null;
if ($overlayEvent.type === OverlayType.POLYGONE) {
const newPolygon = $overlayEvent.overlay.getPath()
.getArray()
.map((latLng: LatLng) => ({ lat: latLng.lat(), lng: latLng.lng() }))
// start and end point should be same for valid geojson
const startPoint = newPolygon[0];
newPolygon.push(startPoint);
$overlayEvent.overlay.setMap(null);
this.polygons = [...this.polygons, newPolygon];
}
}
onClickPolygon(index: number) {
this.activePolygonIndex = index;
}
onEditPolygon(index: number) {
const allPolygons = this.polygonRefs.toArray();
allPolygons[index].getPath()
.then((path: Array<LatLng>) => {
this.polygons[index] = path.map((latLng: LatLng) => ({
lat: latLng.lat(),
lng: latLng.lng()
}))
})
}
onDeleteDrawing() {
this.polygons = this.polygons.filter((polygon, index) => index !== this.activePolygonIndex)
}
}
10. Open app.component.html and add this code.
<h1 class="text-center">Angular Google Maps Drawing Manager</h1>
<div [style.position]="'relative'">
<div
*ngIf="polygons.length"
(click)="onDeleteDrawing()"
[ngStyle]="deleteIconStyle"
title='Delete shape'>
</div>
<agm-map
[latitude]="lat"
[longitude]="lng"
[zoom]="zoom"
[disableDefaultUI]="false"
[zoomControl]="false"
(mapReady)="onLoadMap($event)"
[agmDrawingManager]="drawing">
<agm-drawing-manager
#drawing="agmDrawingManager"
[drawingMode]="drawingMode"
(overlayComplete)="onOverlayComplete($event)"
[drawingControlOptions]="drawingControlOptions"
[polygonOptions]="polygonOptions">
</agm-drawing-manager>
<agm-polygon
*ngFor="let iterator of polygons; index as i"
(polyMouseDown)="onClickPolygon(i)"
(polyMouseUp)="onEditPolygon(i)"
(polyDragEnd)="onEditPolygon(i)"
[fillOpacity]="polygonOptions.fillOpacity"
[fillColor]="polygonOptions.fillColor"
[strokeColor]="polygonOptions.strokeColor"
[strokeWeight]="polygonOptions.strokeWeight"
[polyDraggable]="polygonOptions.draggable"
[editable]="polygonOptions.editable"
[paths]="iterator">
</agm-polygon>
</agm-map>
</div>
11. Finally run the project.
ng serve --o
it will open http://localhost:4200 in the browser.
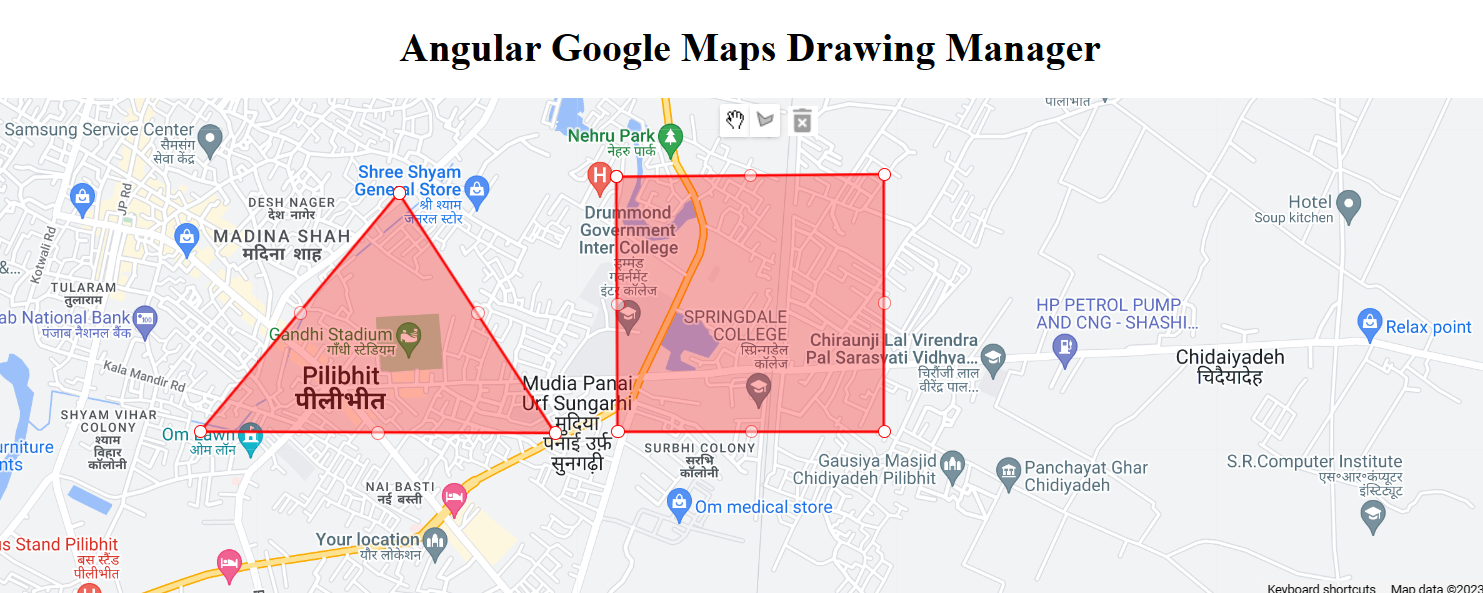
Checkout my full angular-google-maps-drawing-manager example.
https://github.com/ultimateakash/angular-google-maps-drawing-manager
If you facing any issues. don't hesitate to comment below. I will be happy to help you.
Thanks.
Leave Your Comment